Microsoft Intermediate Language – Ultimate Guidance!
In the dynamic world of software development, understanding the intricacies of programming languages and their underlying mechanisms is paramount
Microsoft Intermediate Language (MSIL) is a crucial intermediary language in the .NET Framework, facilitating platform-independent execution of .NET applications.
Join us as we unravel the mysteries of MSIL, empowering developers to harness its potential and elevate their understanding of the .NET ecosystem.
Introduction To Microsoft Intermediate Language (MSIL):
MSIL, or Common Intermediate Language (CIL) or Intermediate Language (IL), is the standard language for .NET applications.
It is a platform-independent, low-level language that acts as a bridge between high-level source code and machine code.
Importance Of Msil In .Net Framework):
The utilization of MSIL is integral to the .NET Framework’s architecture. When a .NET language such as C# or Visual Basic is compiled, it generates MSIL rather than native machine code.
This MSIL code is then executed by the Common Language Runtime (CLR) to produce the desired output.
Understanding The Compilation Process):
1. Source Code To Msil:
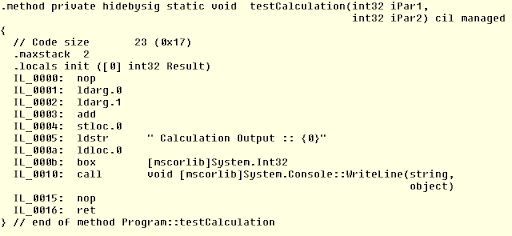
During compilation, source code written in languages supported by the .NET Framework transforms MSIL.
This process retains the structure and logic of the original code while making it compatible with the CLR.
2. Just-In-Time (Jit) Compilation:
Upon execution, MSIL code is not directly executed by the processor. Instead, it undergoes Just-In-Time (JIT) compilation, where it is translated into native machine code specific to the underlying hardware. This ensures optimal performance without sacrificing platform independence.
Advantages Of Msil):
1. Platform Independence:
MSIL ensures .NET applications run on any platform supported by CLR, boosting portability. It abstracts hardware specifics, allowing developers to write code without platform concerns.
2. Performance Optimization:
MSIL employs JIT compilation for tailored performance. During runtime, it’s translated into native code, leveraging hardware for efficient execution without sacrificing platform independence.
3. Language Interoperability:
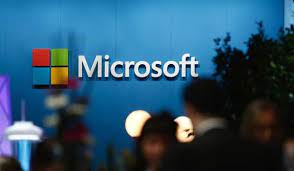
MSIL is a common language for all .NET languages and fosters seamless interoperability. Components in various languages interact effortlessly, promoting code reuse and developer productivity.
4. Debugging And Analysis:
MSIL’s human-readable format aids in analyzing .NET assemblies. Tools like IL Disassembler help debug runtime issues, offering insights for optimization and troubleshooting.
5. Security:
Executing within the CLR sandbox, MSIL undergoes strict security checks. CLR enforces access controls, input validation, and resource protection, mitigating potential security risks.
Common Language Runtime (CLR)):
1. Execution Of Msil Code:
The CLR, as the execution engine of the .NET Framework, plays a pivotal role in running MSIL code.
It provides various services such as memory management, exception handling, and security enforcement to ensure reliable execution.
2. Garbage Collection:
One of the critical features of the CLR is its built-in garbage collector, which automatically manages memory allocation and deallocation. This helps prevent memory leaks and enhances the stability of .NET applications.
Tools For Working With Msil):
1. IL Disassembler (ildasm.exe):
IL Disassembler is a tool provided by the .NET Framework SDK that allows developers to view the MSIL code generated by .NET assemblies.
It provides a human-readable representation of compiled code, aiding in understanding the inner workings of applications.
Developers can use IL Disassembler to debug runtime issues, analyze performance, and gain insights into application behavior.
2. IL Assembler (ilasm.exe):
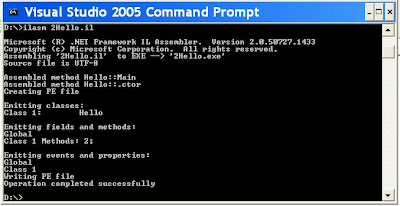
IL Assembler enables developers to L code or modify existing assemblies manually. While less commonly used than other development tools, IL Assembler provides flexibility for advanced scenarios.
Developers proficient in MSIL can use IL Assembler to optimize code, implement custom functionality, or integrate with low-level system components.
Examples Of Msil Code):
1. Basic Arithmetic Operations:
il
Copy code
.method public static int32 Add(int32 a, int32 b)
{
ldarg.0 // Load argument 0 (a)
ldarg.1 // Load argument 1 (b)
add // Add the two values
ret // Return the result
}
This example defines a method named Add, which takes two integers (a and b) as arguments. The extensive instructions load the values of a and b onto the evaluation stack. The add instruction adds these values, and the ret instruction returns the result.
2. Control Flow Statements:
il
Copy code
.method public static int32 Max(int32 a, int32 b)
{
ldarg.0 // Load argument 0 (a)
ldarg.1 // Load argument 1 (b)
ble.s LessThan // Branch if less than or equal to
ldarg.0 // Load argument 0 (a)
ret // Return a
LessThan:
ldarg.1 // Load argument 1 (b)
ret // Return b
}
In this example, a method named Max is defined to return the maximum of two integers (a and b). The extensive instructions load the values of a and b onto the evaluation stack.
The ble.s instruction branches to the LessThan label if a is less than or equal to b. If the condition is proper, it loads and returns it. Otherwise, it loads b and returns it.
Best Practices For Writing Msil Code):
1. Code Optimization Techniques:
- Minimize unnecessary instructions: Remove redundant or unused instructions to streamline code execution.
- Utilize efficient algorithms: Choose algorithms and data structures that optimize performance and resource utilization.
- Avoid excessive branching: Minimize conditional branches and loops to reduce computational overhead.
- Optimize memory usage: Use memory-efficient data types and structures and minimize unnecessary memory allocations.
2. Security Considerations:
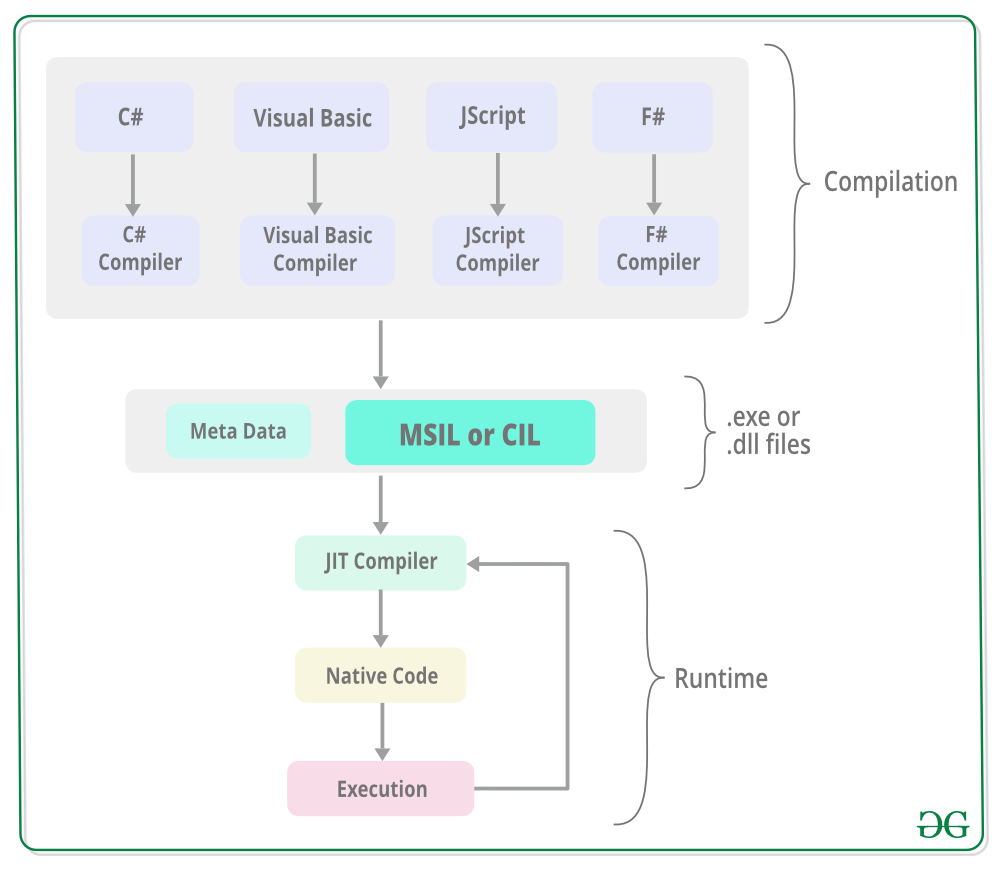
- Validate input: Ensure all input data is validated to prevent security vulnerabilities such as buffer overflows or injection attacks.
- Enforce code access security: Apply appropriate access controls and permissions to restrict unauthorized access to sensitive resources.
- Implement encryption: Use encryption algorithms and protocols to protect sensitive data from unauthorized access or tampering.
3. Consistency And Readability:
- Use descriptive names: Choose meaningful and descriptive identifiers for variables, methods, and classes to enhance code readability.
- Follow naming conventions: Adhere to established naming conventions to maintain consistency and make code easier to understand for other developers.
4. Modularity And Reusability:
- Encapsulate functionality: Organize code into logical modules and classes to promote encapsulation and reusability.
- Favor composition over inheritance: Use composition and interface-based design to create flexible and reusable components.
5. Performance Profiling And Testing:
- Profile code performance: Use profiling tools to identify performance bottlenecks and optimize critical code sections.
- Conduct thorough testing: Test code under various conditions and edge cases to ensure robustness, reliability, and compatibility.
Related Questions:
1. Is Msil The Same As Machine Code?
MSIL is an intermediate language translated into native machine code at runtime by the Common Language Runtime (CLR).
2. Can Msil Code Be Decompiled?
Tools like IL Disassembler allow developers to view the MSIL code of compiled assemblies.
3. Does Writing Msil Directly Offer Performance Benefits?
In most cases, writing MSIL directly offers little performance benefits compared to using high-level languages like C# or Visual Basic. However, it can be helpful in specific optimization scenarios.
4. Can Msil Code Run On Non-Windows Platforms?
Yes, MSIL code can run on any platform supported by the Common Language Runtime (CLR), including Linux and macOS.
5. Is Msil Used Only In .Net Framework?
While MSIL is primarily associated with the .NET Framework, its principles are also utilized in other frameworks like Mono and .NET Core.
Conclusion:
In conclusion, Microsoft Intermediate Language (MSIL) is a vital component of the .NET Framework, bridging the gap between source code and execution. Its platform independence, performance optimization, and language interoperability make it indispensable for modern software development. By understanding MSIL and its role within the .NET ecosystem, developers can unlock new possibilities and enhance the quality of their applications.
Also Read: